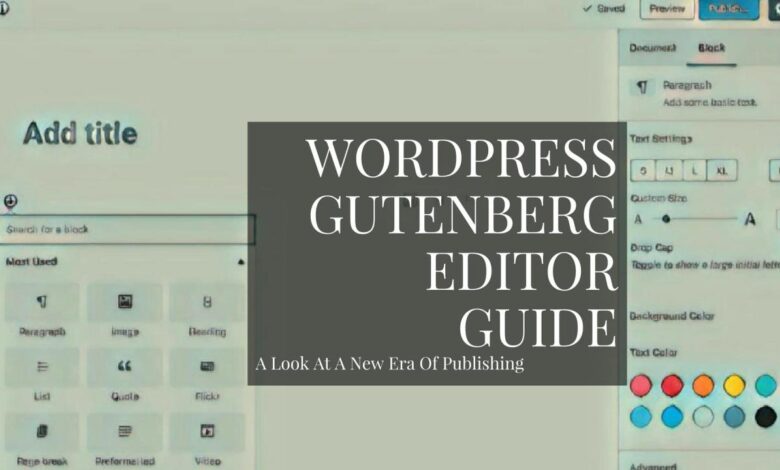
WordPress Gutenberg Plugin Builder A Deep Dive
WordPress Gutenberg plugin builder: Ever wished you could craft custom WordPress blocks with ease? This post dives deep into the world of Gutenberg plugin builders, exploring how they empower you to extend WordPress functionality beyond its default capabilities. We’ll cover everything from building simple image blocks to creating dynamic, data-fetching marvels, all while touching upon best practices for security and performance.
We’ll walk you through the process step-by-step, from choosing the right plugin builder to deploying your custom creations. Whether you’re a seasoned developer or just starting out, this guide offers insights and practical examples to help you master the art of Gutenberg plugin development. Get ready to unlock the full potential of WordPress and create truly unique user experiences!
Introduction to WordPress Gutenberg Plugin Builders
WordPress Gutenberg, the block editor, revolutionized website building. But its power can be significantly enhanced with plugins designed specifically to extend its functionality. These plugins, known as Gutenberg plugin builders, provide tools to create custom blocks, modify existing ones, and even build entire themes using the Gutenberg interface. They streamline the development process and unlock a whole new level of customization for WordPress users, from beginners to seasoned developers.
Using a Gutenberg plugin builder offers several key advantages. Firstly, it significantly speeds up development. Instead of writing code from scratch, you can utilize pre-built components and intuitive interfaces to create blocks and themes. This reduces development time and allows for quicker iteration and experimentation. Secondly, it lowers the barrier to entry for non-developers.
Even those with limited coding experience can create functional and visually appealing blocks and themes. Finally, these builders often provide enhanced functionality, such as improved performance optimization, accessibility features, and compatibility with various WordPress themes.
Types of Gutenberg Plugin Builders
Gutenberg plugin builders come in various forms, each catering to different needs and skill levels. Block builders are specifically designed for creating individual Gutenberg blocks, offering tools to design layouts, add custom functionality, and integrate with other services. Theme builders, on the other hand, allow you to construct entire WordPress themes using the Gutenberg interface, providing a more comprehensive approach to theme development.
Some plugins even combine both functionalities, offering a unified platform for block and theme creation. There are also plugins focused on specific aspects of Gutenberg, like improving its performance or adding advanced styling options.
Comparison of Popular Gutenberg Plugin Builders
The market offers several powerful Gutenberg plugin builders. Choosing the right one depends on your specific needs and budget. Below is a comparison of three popular options:
Name | Key Features | Pricing | Ease of Use |
---|---|---|---|
Elementor | Drag-and-drop interface, extensive library of pre-built blocks and templates, theme building capabilities, WooCommerce integration. | Free and Pro versions (Pro version offers advanced features) | Very easy, suitable for beginners. |
Beaver Builder | Intuitive drag-and-drop interface, responsive design tools, pre-built templates, theme building capabilities, extensive customization options. | Free and Pro versions (Pro version unlocks more features and templates). | Easy to learn, good for beginners and intermediate users. |
Gutenberg Blocks (Core WordPress functionality) | Basic block creation and editing capabilities, extensible via additional plugins. | Free (included with WordPress) | Moderate; requires some familiarity with WordPress and block editing. |
Building a Simple Gutenberg Block with a Plugin Builder
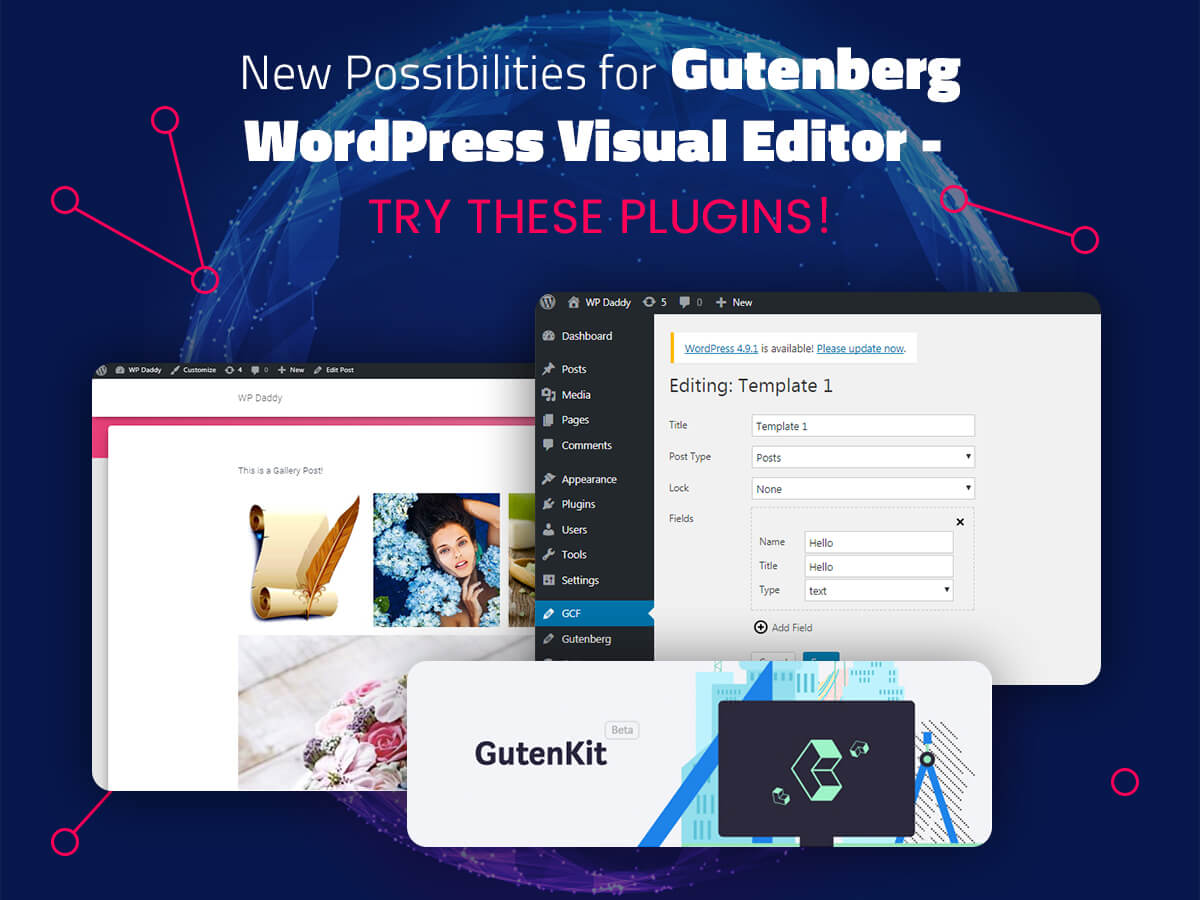
Source: wpdaddy.com
Creating custom Gutenberg blocks significantly enhances the WordPress editing experience, allowing developers to provide users with rich, reusable content elements. This process is greatly simplified through the use of plugin builders, which handle much of the boilerplate code and configuration. Let’s explore building a basic block using this approach. We’ll focus on a simple image block with a caption, illustrating the core concepts involved.
Creating a Basic Image Block
We’ll use a hypothetical plugin builder (the specific steps might vary slightly depending on the builder you choose). The general process usually involves defining the block’s attributes, rendering its front-end output, and creating the editor interface. The builder often provides a user-friendly interface to handle this, reducing the need for extensive manual coding. The core concept remains consistent across various builders.First, we define the block’s attributes.
These attributes determine the data the block will hold, such as the image URL and caption text. This is typically done within the block’s registration function. For our image block, we need at least two attributes: `imageUrl` (string) and `caption` (string).
Implementing Block Attributes and Controls
Within the plugin builder, you’ll usually find a section to define attributes. You’d add an attribute named `imageUrl` of type string and another named `caption`, also a string. This defines the data structure of our block.Next, we create the front-end rendering function. This function takes the block’s attributes as input and generates the HTML output. For our simple image block, this might look like this (the exact syntax will depend on the plugin builder):“`javascriptfunction edit( attributes, setAttributes ) const imageUrl, caption = attributes; return (
caption
);function save( attributes ) const imageUrl, caption = attributes; return (
caption
);“`This code renders an image tag using the `imageUrl` attribute and a paragraph tag for the caption. The `alt` attribute of the image tag uses the caption for accessibility. The `save` function is responsible for the final output on the frontend. It mirrors the `edit` function in this simple example.
Adding Editor Controls
To allow users to easily input the image URL and caption, we need to add controls to the block’s editor interface. Most plugin builders provide a visual interface or code snippets to add these controls. You’d typically add a media uploader for the image and a text input for the caption. This would be within the `edit` function.
For example:“`javascriptfunction edit( attributes, setAttributes ) const imageUrl, caption = attributes; return (
caption
);“`This adds a “Choose Image” button that opens the WordPress media library and a text input for the caption. The values are dynamically updated using the `setAttributes` function.
Handling Block Variations
While our example is simple, more complex blocks might require variations. For example, you might want to offer different image sizes or alignment options. Plugin builders often offer mechanisms to handle variations, either through a visual interface or by extending the block’s registration function. This involves defining different attribute combinations and corresponding rendering logic. For instance, you could add an `alignment` attribute to control image alignment (left, center, right).
You would then use conditional rendering in your `edit` and `save` functions to display the image accordingly. This enhances the block’s flexibility and caters to diverse user needs.
Advanced Gutenberg Block Development Techniques
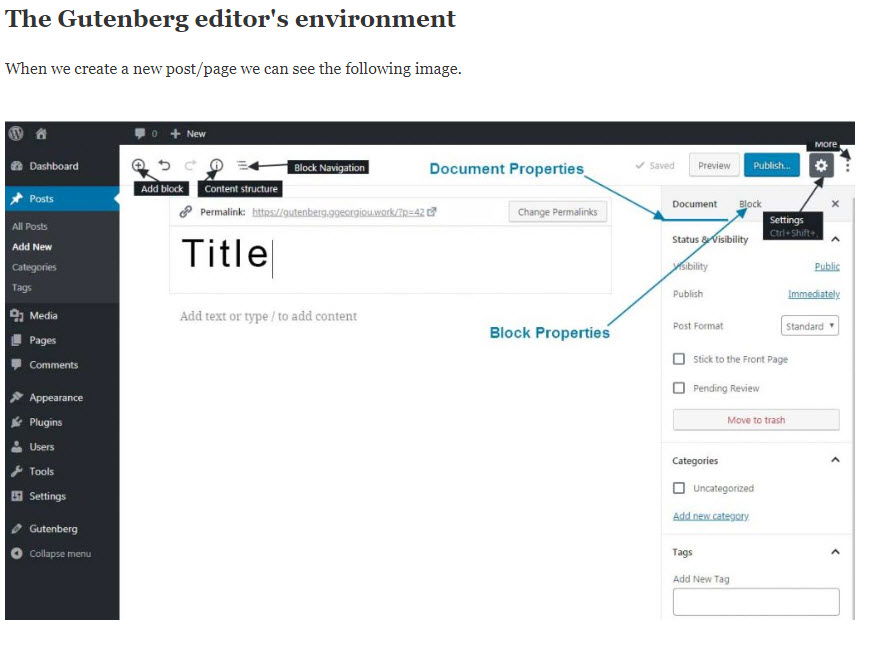
Source: wprepublic.com
Building basic Gutenberg blocks is a great start, but to truly harness the power of this editor, you need to delve into more advanced techniques. This section explores strategies for creating dynamic, performant, and maintainable blocks that go beyond the fundamentals. We’ll cover fetching data from external APIs, implementing server-side rendering, and building blocks that interact with your custom database.
Fetching Data from External APIs, WordPress gutenberg plugin builder
Integrating external data sources significantly enhances your Gutenberg blocks’ functionality. Imagine a block displaying real-time stock prices, weather updates, or news feeds – all dynamically updated without page refreshes. This is achieved by making API calls within your block’s JavaScript code. The process generally involves fetching data using `fetch` or a similar library, parsing the JSON response, and then rendering that data within the block’s interface.
Error handling and loading indicators are crucial for a smooth user experience. For example, you might use a try-catch block to handle potential network errors and display a user-friendly message if the API request fails. Furthermore, you can implement a loading spinner while the data is being fetched to provide visual feedback to the user.
Server-Side Rendering for Improved Performance
Server-side rendering (SSR) is a game-changer for Gutenberg block performance, especially when dealing with complex or data-intensive blocks. Instead of rendering the entire block client-side, SSR generates the block’s HTML on the server, sending only the rendered output to the browser. This reduces the load on the client and improves initial load times, leading to a faster and more responsive website.
WordPress provides mechanisms like the `render_callback` function within your block’s registration to enable SSR. This function handles the server-side rendering logic, allowing you to prepare the block’s content before it’s sent to the client. This approach is particularly beneficial for blocks that require significant processing or database queries, as these tasks are handled on the server, leaving the client with less work to do.
Best Practices for Efficient and Maintainable Code
Writing clean, well-structured code is essential for creating maintainable Gutenberg blocks. This includes using a consistent coding style, employing meaningful variable names, and adding comprehensive comments to explain complex logic. Leveraging component-based architecture, where you break down your block into smaller, reusable components, improves code organization and reusability. Furthermore, using version control (like Git) is critical for tracking changes and collaborating effectively on block development.
Adopting a testing strategy, including unit and integration tests, helps ensure the block’s functionality and prevents regressions. Remember to utilize linters and code formatters to maintain consistency and identify potential issues early on.
Creating a Gutenberg Block Using a Custom Database Table
This involves several steps. First, you need to create the custom database table using SQL. This table will store the data your block will use. Next, you’ll create functions to interact with this table, including functions to insert, update, retrieve, and delete data. These functions will use the WordPress database API.
Within your Gutenberg block’s JavaScript, you’ll make AJAX calls to these server-side functions to fetch and manipulate data. Finally, you’ll render the fetched data within the block’s interface. For instance, you might create a custom post type to manage entries related to your custom database table. This could be for managing products, events, or any other data that your block needs to display.
The block would then fetch data from this custom post type, dynamically displaying the information within the Gutenberg editor. This structured approach ensures data integrity and facilitates efficient management of the data used by your block.
Integrating Gutenberg Blocks with WordPress Themes
Seamlessly integrating custom Gutenberg blocks into your WordPress theme elevates the site-building experience, allowing users to easily incorporate your custom content elements. This integration requires careful consideration of how your blocks interact with your theme’s structure and styling. Proper registration and enqueuing of scripts and styles are crucial for functionality and visual consistency.Integrating custom blocks involves registering them within your theme’s functions.php file or a custom plugin dedicated to your theme’s functionality.
This ensures the blocks are available for use within the Gutenberg editor. The method of integration into theme templates then allows for dynamic content placement and manipulation within your site’s layout.
Registering and Enqueuing Block Assets
To ensure your custom blocks function correctly and appear visually appealing, you must register and enqueue the necessary JavaScript and CSS files. This is done using WordPress’s built-in functions. Failing to properly enqueue assets will result in broken or poorly styled blocks. The process typically involves using `wp_register_script`, `wp_enqueue_script`, `wp_register_style`, and `wp_enqueue_style` functions within a function hooked to the `enqueue_block_editor_assets` action.
This ensures the assets are only loaded in the Gutenberg editor. For frontend assets, use `wp_enqueue_scripts`.
Incorporating Blocks into Theme Templates
There are several ways to incorporate custom Gutenberg blocks into your theme templates. The most straightforward method involves using the `has_block()` function to check for the presence of a specific block within the post content. If the block exists, you can then render its output using the appropriate template tags. This allows for dynamic and flexible content placement within your theme’s structure.
Alternatively, you can use a shortcode, enabling users to insert the block into a specific area of your template using a simple shortcode. This method is less flexible but can be easier to implement for less technical users.
Code Example: Using a Custom Block in a Theme’s Single Post Template
Let’s assume we have a custom Gutenberg block named “advanced-quote” that renders a styled quote. To use this block within our theme’s `single.php` template (the template responsible for displaying individual posts), we can use the following code:“`php Custom Quotes from this Post:
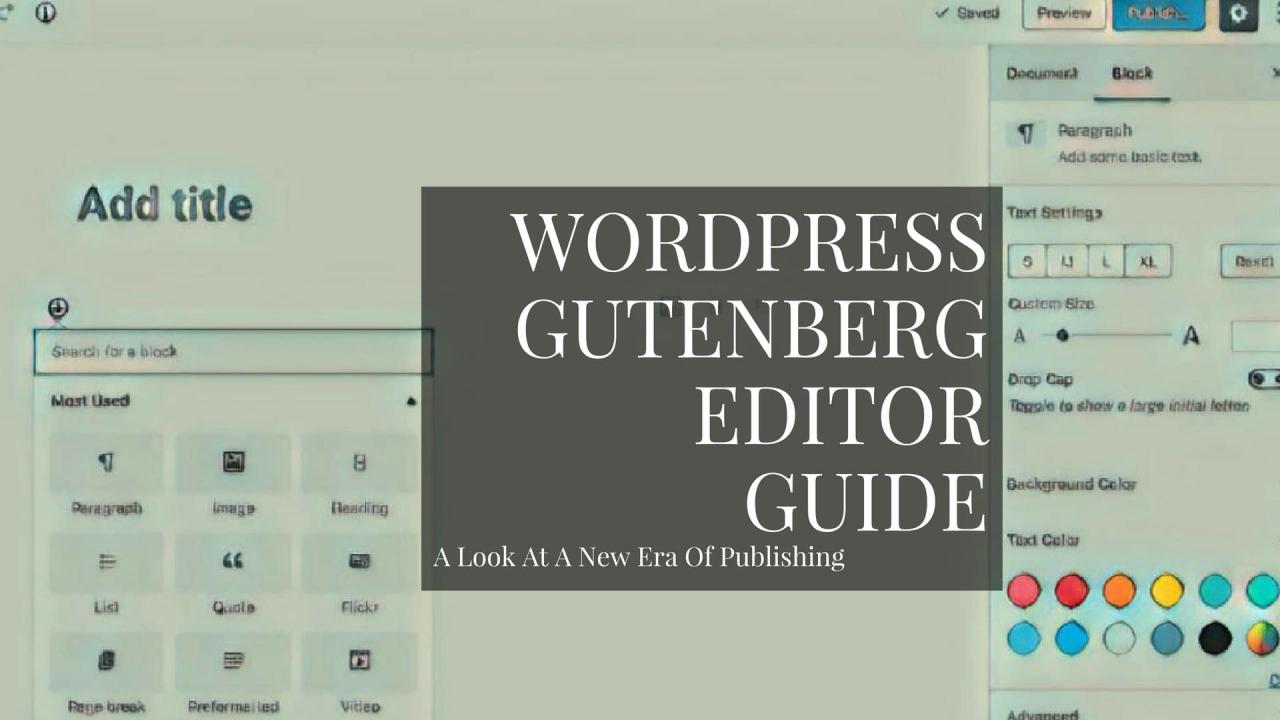
Source: gutenberghub.com
‘; while ( have_posts() ) : the_post(); the_content(); endwhile;?>“`This code snippet checks if the `my-plugin/advanced-quote` block exists within the current post.
If it does, it will output the content of that block within the `single.php` template. This allows you to integrate your custom blocks into specific areas of your theme’s layout based on their presence within a post’s content. Remember to replace `my-plugin/advanced-quote` with the actual namespace and name of your custom block. This example assumes the block handles its own output; more complex blocks might require additional logic for data retrieval and presentation.
Troubleshooting and Debugging Gutenberg Plugin Development
Developing Gutenberg plugins can be rewarding, but it’s not always smooth sailing. Expect to encounter unexpected errors and challenges along the way. This section focuses on identifying common problems, providing practical debugging strategies, and ensuring your blocks work seamlessly across various platforms. Mastering these techniques will significantly improve your workflow and the quality of your plugins.
Common Issues in Gutenberg Plugin Development
Debugging Gutenberg plugins often involves JavaScript errors, conflicts with other plugins, and inconsistencies across different browsers and devices. These issues can manifest in various ways, from blocks failing to render correctly to unexpected behavior within the editor. Understanding these common pitfalls is the first step toward effective troubleshooting. For instance, a common problem is incorrect usage of React lifecycle methods, leading to rendering errors or unexpected state updates.
Another frequent issue stems from improper handling of data attributes, which can result in blocks not functioning as intended. Finally, failing to consider browser compatibility can lead to blocks rendering differently or malfunctioning entirely across different browsers.
Debugging JavaScript Errors
JavaScript errors are a frequent headache in Gutenberg development. The browser’s developer console is your best friend here. It provides detailed error messages, including line numbers and stack traces, pinpointing the source of the problem. Leverage browser developer tools to set breakpoints in your code, step through execution line by line, and inspect variable values. Employing a linter, such as ESLint, can also help catch potential errors early in the development process.
This prevents many issues before they even reach the browser. For instance, a forgotten semicolon or an incorrect variable declaration can be identified and corrected before they cause a runtime error.
Resolving Conflicts with Other Plugins
Conflicts with other plugins can be tricky to diagnose. Start by deactivating other plugins one by one to isolate the source of the conflict. If a specific plugin is identified, check its documentation for potential compatibility issues. If the conflict persists, examine the code of both plugins for overlapping functionality or conflicting JavaScript libraries. Using a debugging tool that allows for stepping through the execution of both plugins simultaneously can be helpful in pinpointing the precise point of conflict.
For example, a conflict might arise if two plugins attempt to register the same block name or utilize the same JavaScript library version, causing unexpected behavior.
Testing Gutenberg Blocks Across Different Browsers and Devices
Thorough testing is crucial for ensuring your Gutenberg blocks function correctly across various browsers and devices. Test on popular browsers like Chrome, Firefox, Safari, and Edge. Use browser developer tools to simulate different screen sizes and resolutions to check responsiveness. Consider using automated testing frameworks to streamline the testing process and catch regressions early. A simple approach could be manual testing across different browsers and device emulators.
However, for larger projects, employing a framework like Cypress or Selenium could significantly improve the efficiency and coverage of your testing process. This is essential to guarantee consistent user experience across different platforms.
Troubleshooting Flowchart for Gutenberg Block Rendering Issues
The following flowchart Artikels a systematic approach to resolving common rendering issues:
Step | Action | Outcome |
---|---|---|
1 | Check the browser’s developer console for JavaScript errors. | Identifies and resolves JavaScript errors. |
2 | Inspect the block’s HTML structure using the browser’s developer tools. | Reveals structural issues in the rendered block. |
3 | Deactivate other plugins to rule out conflicts. | Identifies potential plugin conflicts. |
4 | Test on different browsers and devices. | Determines browser-specific issues. |
5 | Check the block’s code for syntax errors and logical inconsistencies. | Fixes errors in the block’s code. |
6 | Verify that the necessary dependencies are correctly included and loaded. | Ensures proper functionality of the block. |
Extending Gutenberg Functionality
Gutenberg’s extensibility is a key feature, allowing developers to tailor the editor to their specific needs. This goes beyond simply creating custom blocks; it involves modifying the core editor’s behavior and adding features that integrate seamlessly with the existing workflow. This section explores powerful techniques for extending Gutenberg’s capabilities through plugin development.Extending Gutenberg functionality primarily involves leveraging the various hooks and filters WordPress provides, combined with a deep understanding of Gutenberg’s internal architecture.
This allows for customization without directly modifying core files, ensuring updates remain smooth and conflict-free. The power lies in strategically intercepting and modifying data flow within the editor.
Custom Gutenberg Block Categories
Creating custom categories helps organize blocks logically, improving user experience and discoverability. This is achieved using the `register_block_type` function, specifically the `category` argument. For instance, a plugin adding blocks for e-commerce functionality might register its blocks under a category named ‘ecommerce’. This category will then appear in the block inserter, grouping related blocks together. The code would look something like this: register_block_type( 'my-plugin/my-ecommerce-block', [ 'category' => 'ecommerce', // ... other block settings] );
This simple addition significantly enhances the user interface, making it easier for users to find and utilize the custom blocks.
Custom Gutenberg Block Panels
Custom panels provide an organized way to present additional settings and options within a block’s sidebar. This involves utilizing the `register_block_type` function and the `attributes` property in conjunction with the `InspectorControls` component within the block’s edit function. The `InspectorControls` component renders the custom panel in the sidebar. For example, a custom block might have a panel for advanced styling options.
These options are defined as attributes within the block definition and rendered within the `InspectorControls` component using React components.
Building WordPress plugins with Gutenberg’s block editor is a fantastic way to extend functionality, and honestly, it’s made my workflow so much smoother. I’ve been focusing lately on improving my plugin’s video integration, which led me to check out some great tips on getting it on with YouTube for better video marketing. The knowledge gained there directly improved how I’m handling video embeds within my WordPress Gutenberg plugin builder project, making the whole experience much more user-friendly.
Adding Custom Block Attributes and Controls
Extending block functionality often necessitates custom attributes to store additional data. These attributes are then associated with controls within the block’s settings panel. This is done through the `attributes` property in `register_block_type`. For example, adding a background color attribute would involve defining it within the `attributes` array and creating a color picker control within `InspectorControls` to modify it. attributes: backgroundColor: type: 'string', default: '#ffffff' ,
The corresponding control within the `InspectorControls` would use a React component like `ColorPalette` to allow users to select the background color.
Integrating Gutenberg Blocks with a Popular WordPress Plugin
Consider integrating a custom Gutenberg block with WooCommerce, a popular e-commerce plugin. This might involve creating a block that displays a product carousel. This would require using the WooCommerce REST API to fetch product data. The block would then use this data to render the carousel. This integration leverages both Gutenberg’s block development capabilities and the external plugin’s API.
The block would need to fetch product data using `wp.apiFetch` (or a similar method) and then display it using React components. Error handling and efficient data management are crucial aspects of this type of integration. A well-structured approach would involve separate components for fetching data, handling loading states, and rendering the carousel itself, promoting code maintainability and reusability.
Security Considerations for Gutenberg Plugins: WordPress Gutenberg Plugin Builder
Building secure Gutenberg plugins is crucial to protect your users and your website. Neglecting security can lead to vulnerabilities that malicious actors can exploit, resulting in data breaches, website defacement, or even complete server compromise. This section will Artikel key security considerations and best practices to help you develop robust and secure plugins.
Potential Security Vulnerabilities and Mitigation Strategies
Several vulnerabilities can arise in Gutenberg plugins if not properly addressed. Cross-Site Scripting (XSS) attacks, for example, occur when unsanitized user input is directly outputted to the browser, allowing attackers to inject malicious JavaScript code. SQL injection vulnerabilities can occur if user input is directly used in database queries without proper escaping or parameterized queries. Finally, insecure file handling, such as allowing users to upload arbitrary files without validation, can lead to the execution of malicious code or the compromise of sensitive data.
Mitigation strategies involve robust input validation and sanitization, using prepared statements for database interactions, and carefully controlling file uploads with strict validation and type checking. Employing an established security framework and adhering to coding best practices are also vital.
Data Sanitization and Validation Best Practices
Data sanitization and validation are paramount for secure Gutenberg plugin development. Input sanitization removes or neutralizes potentially harmful characters or code from user-supplied data before it’s processed or stored. Validation ensures that the data conforms to expected formats and constraints. For example, if a block accepts a URL, validating that it’s a correctly formatted URL and sanitizing it to prevent injection attacks is essential.
Similarly, if a block accepts numerical data, validating that it’s a number and sanitizing it to prevent SQL injection is crucial. Consistent and thorough application of these practices across all data handling processes significantly reduces the risk of security vulnerabilities. This involves not only sanitizing user input, but also data retrieved from the database before it’s displayed to the user.
Input Sanitization and Validation in Gutenberg Plugin Development
WordPress provides several functions to assist with input sanitization and validation. `sanitize_text_field()` removes harmful HTML tags and characters from text, `esc_url()` sanitizes URLs, and `absint()` ensures that a value is a non-negative integer. These functions should be used extensively when handling user input. Furthermore, using WordPress’s built-in validation functions, along with custom validation rules where necessary, helps to maintain data integrity and prevent malicious inputs from affecting the plugin’s functionality or the WordPress site itself.
For example, a custom validation function could check if an uploaded image file is of a specific type and size before it’s processed. Failure to utilize these functions increases the likelihood of vulnerabilities.
Security Checklist for Gutenberg Plugins
Before releasing any Gutenberg plugin, a comprehensive security checklist should be followed. This checklist should include:
- Input Validation and Sanitization: Verify that all user input is validated and sanitized using appropriate WordPress functions.
- Output Escaping: Ensure that all data outputted to the browser is properly escaped to prevent XSS attacks.
- Database Interactions: Use prepared statements or parameterized queries to prevent SQL injection vulnerabilities.
- File Handling: Implement strict validation and sanitization for uploaded files to prevent malicious code execution.
- Authentication and Authorization: If the plugin handles sensitive data, implement robust authentication and authorization mechanisms.
- Regular Security Audits: Conduct regular security audits to identify and address potential vulnerabilities.
- Code Reviews: Conduct thorough code reviews to identify potential security flaws before release.
- Use of Established Security Frameworks: Consider using established security frameworks to guide your development process.
Following this checklist significantly reduces the likelihood of security vulnerabilities in your Gutenberg plugins. Regular updates and patching of identified vulnerabilities are also critical aspects of maintaining a secure plugin.
Outcome Summary
Building custom Gutenberg blocks opens a world of possibilities for enhancing your WordPress sites. By leveraging the power of plugin builders, you can efficiently create tailored blocks, boosting both functionality and user engagement. Remember to prioritize security best practices and thorough testing throughout the development process. So go forth, experiment, and build amazing things! Happy coding!
Helpful Answers
What are the limitations of using a Gutenberg plugin builder?
While plugin builders simplify development, they might have limitations in terms of highly customized functionalities. Very complex blocks might require more manual coding.
How do I choose the right Gutenberg plugin builder for my needs?
Consider factors like your coding experience, the complexity of your blocks, the builder’s features, and its pricing. Start with a free option if you’re unsure.
Are there any performance considerations when using custom Gutenberg blocks?
Yes, avoid overly complex blocks or those that load large amounts of unnecessary data. Server-side rendering can significantly improve performance.
Where can I find more resources to learn about Gutenberg plugin development?
The official WordPress developer resources, along with numerous online tutorials and courses, are excellent starting points.