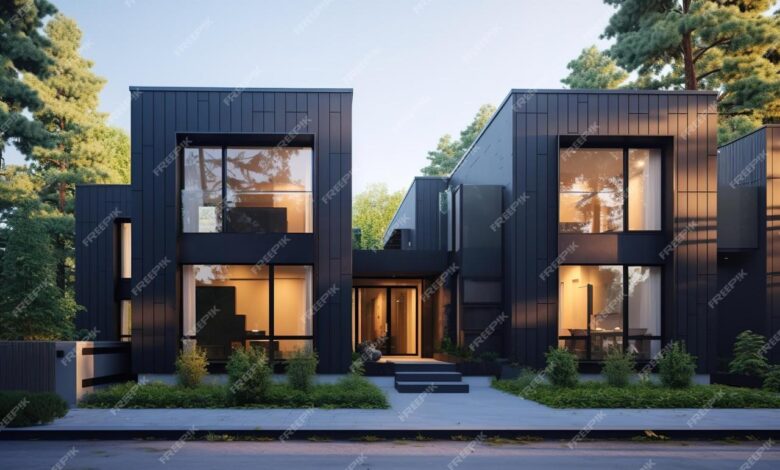
Decorators with a Difference Beyond the Basics
Decorators with a difference: Dive into the fascinating world of Python decorators, moving beyond the simple examples often presented. We’ll explore advanced techniques, uncover hidden potential, and discover how decorators can dramatically improve your code’s elegance, efficiency, and maintainability. This isn’t your grandma’s decorator tutorial; we’re venturing into the realm of decorator factories, dynamic argument handling, and even the powerful synergy between decorators and metaclasses.
Prepare to master the art of creating decorators that handle multiple arguments, efficiently chain together for complex functionalities, and even measure the execution time of your functions. We’ll tackle real-world applications like logging, input validation, and caching, showcasing how decorators can transform your code from messy to magnificent. Get ready to unlock a new level of Python proficiency!
Decorators Beyond the Basics
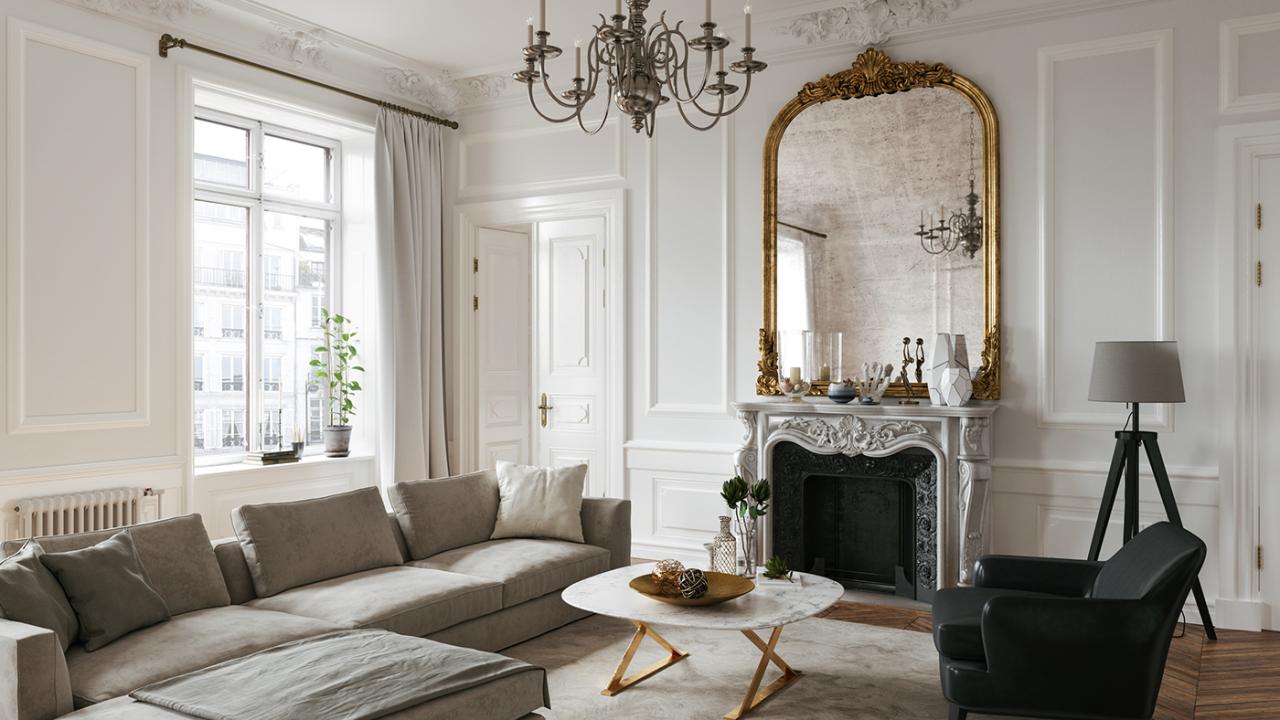
Source: modernfloorlamps.net
Decorators are a powerful feature in Python that allow you to modify or enhance functions and methods in a clean and readable way. They essentially wrap additional functionality around an existing function without modifying its core behavior. Think of them as a way to add extra layers of functionality like sprinkles on a cupcake – the cupcake (your function) is still the same, but it’s been improved.Decorators are frequently used for tasks like logging, access control, instrumentation (measuring performance), and caching.
By using decorators, you keep your core code concise and focused, improving overall code maintainability and readability. Instead of repeating the same code blocks across multiple functions, you encapsulate them within a decorator, applying them as needed.
Simple Decorator Examples
Let’s start with a couple of basic examples to illustrate the concept. A simple decorator might add logging to a function:“`pythonimport functoolsdef my_decorator(func): @functools.wraps(func) #Preserves function metadata def wrapper(*args,
*kwargs)
print(f”Calling function: func.__name__”) result = func(*args, – *kwargs) print(f”Function func.__name__ finished.”) return result return wrapper@my_decoratordef say_hello(name): print(f”Hello, name!”) return f”Hello, name!”say_hello(“World”)“`This decorator, `my_decorator`, prints messages before and after the execution of the `say_hello` function.
The `@functools.wraps` decorator is crucial here; it ensures that metadata (like the function’s name and docstring) is preserved after decoration.Another example could be a decorator that measures the execution time of a function:“`pythonimport timeimport functoolsdef time_it(func): @functools.wraps(func) def wrapper(*args,
*kwargs)
start_time = time.time() result = func(*args, – *kwargs) end_time = time.time() print(f”Execution time of func.__name__: end_time – start_time:.4f seconds”) return result return wrapper@time_itdef slow_function(): time.sleep(1) return “Slow function finished”slow_function()“`Here, the `time_it` decorator measures and prints the execution time of the `slow_function`.
This is incredibly useful for performance analysis and optimization. Note again the use of `functools.wraps` to maintain the original function’s information.
Advanced Decorator Techniques: Decorators With A Difference
So far, we’ve covered the basics of decorators, but the real power lies in mastering more advanced techniques. This section delves into decorator factories, dynamically handling multiple arguments, chaining decorators effectively, and measuring function execution times – essential skills for any Python developer looking to write clean, efficient, and reusable code.
Decorator Factories
Decorator factories are functions that return decorators. This allows for the creation of decorators that can be customized at runtime. Consider a scenario where you need to log different messages depending on the function being decorated. A simple decorator wouldn’t suffice. A decorator factory solves this elegantly.
Here’s how you might implement one:“`pythondef log_decorator_factory(log_message): def decorator(func): def wrapper(*args,
*kwargs)
print(f”Before func.__name__: log_message”) result = func(*args, – *kwargs) print(f”After func.__name__: log_message”) return result return wrapper return decorator@log_decorator_factory(“Starting operation…”)def my_function(): print(“Inside my_function”)my_function()“`This example creates a `log_decorator_factory` that takes a log message as input and returns a decorator.
Each function decorated with the factory’s output will have its execution logged with the specified message. This provides flexibility and reusability without repeating code.
Dynamically Handling Multiple Arguments
Decorators are often designed to work with functions that have specific argument signatures. However, creating a decorator that can handle any number of arguments, both positional and , requires a more sophisticated approach. This is crucial when dealing with functions of unknown or varying input.“`pythondef dynamic_decorator(func): def wrapper(*args,
*kwargs)
print(f”Function func.__name__ called with args: args, kwargs: kwargs”) return func(*args, – *kwargs) return wrapper@dynamic_decoratordef my_flexible_function(a, b, c=3): return a + b + cprint(my_flexible_function(1,2))print(my_flexible_function(1,2,c=4))“`The `dynamic_decorator` utilizes `*args` and `kwargs` to accept any number of positional and arguments, making it adaptable to various function signatures.
This approach enhances the versatility and reusability of decorators.
Chaining Decorators
Chaining decorators involves applying multiple decorators to a single function. The order of application is crucial, as each decorator wraps the result of the previous one. Consider the following example illustrating both simple and nested chaining:“`pythondef bold_decorator(func): def wrapper(*args,
*kwargs)
return f” func(*args, – *kwargs)” return wrapperdef italic_decorator(func): def wrapper(*args,
*kwargs)
return f” func(*args, – *kwargs)” return wrapper@bold_decorator@italic_decoratordef my_text(): return “Hello, world!”print(my_text()) # Output: Hello, world!# Nested approach (equivalent)decorated_function = bold_decorator(italic_decorator(my_text))print(decorated_function()) # Output: Hello, world!“`The example demonstrates that applying `@bold_decorator` after `@italic_decorator` results in the text being italicized first, then bolded. The order directly impacts the final output.
The nested approach explicitly shows the function wrapping process.
Measuring Function Execution Time
Measuring the execution time of a function is a common task, particularly during performance optimization. A decorator can elegantly handle this:“`pythonimport timedef execution_time_decorator(func): def wrapper(*args,
*kwargs)
start_time = time.time() result = func(*args, – *kwargs) end_time = time.time() execution_time = end_time – start_time print(f”Function func.__name__ took execution_time:.4f seconds to execute.”) return result return wrapper@execution_time_decoratordef time_consuming_function(): time.sleep(1) return “Task completed”time_consuming_function()“`This decorator uses the `time` module to calculate the difference between the start and end times of the function’s execution, providing a precise measurement of its performance.
This is invaluable for identifying performance bottlenecks in your applications.
Decorators for Specific Use Cases
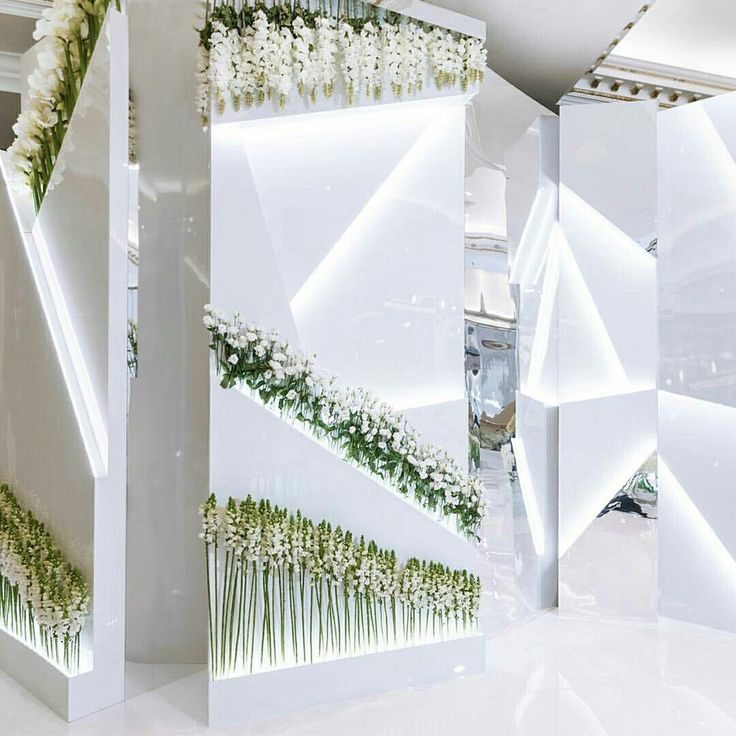
Source: pinimg.com
Decorators are a powerful tool in Python, allowing us to modify or enhance functions and methods in a clean and readable way. Beyond the basic examples, decorators can be tailored to solve specific problems, improving code efficiency, maintainability, and robustness. This section explores the implementation of decorators for common use cases, showcasing their versatility and practical applications.
Logging Function Calls
A logging decorator can significantly aid in debugging and monitoring applications. It adds functionality to record details about function calls, such as the function’s name, arguments passed, return value, and execution time. This information is invaluable for tracking down errors or analyzing application performance. Here’s an example implementation:“`pythonimport functoolsimport loggingimport timedef log_calls(func): @functools.wraps(func) # Preserve original function metadata def wrapper(*args,
*kwargs)
logger = logging.getLogger(__name__) start_time = time.time() logger.info(f”Calling function: func.__name__ with args: args, kwargs: kwargs”) result = func(*args, – *kwargs) end_time = time.time() logger.info(f”Function func.__name__ returned: result in end_time – start_time:.4f seconds”) return result return wrapper# Example usagelogging.basicConfig(level=logging.INFO)@log_callsdef my_function(a, b): time.sleep(1) # Simulate some work return a + bmy_function(2, 3)“`This decorator uses the `logging` module to record messages at the INFO level.
The `functools.wraps` decorator ensures that the wrapper function retains the original function’s metadata, preventing issues with introspection.
Input Validation
Ensuring the validity of input data is crucial for preventing errors and maintaining application stability. A decorator can enforce input validation before a function executes, handling invalid inputs gracefully.“`pythondef validate_input(func): @functools.wraps(func) def wrapper(*args,
*kwargs)
# Example validation: check if arguments are integers and positive for arg in args: if not isinstance(arg, int) or arg <= 0: raise ValueError("Input must be positive integers.") return func(*args, -*kwargs) return wrapper @validate_input def my_calculation(x, y): return x - y print(my_calculation(5, 10)) # Valid input #print(my_calculation(-5, 10)) # Raises ValueError ``` This decorator checks if the input arguments are positive integers. If not, it raises a `ValueError`. More sophisticated validation can be implemented, adapting to specific data types and constraints.
Caching Results of Computationally Expensive Functions
For functions with high computational costs, caching their results can dramatically improve performance.
A caching decorator stores the results of previous function calls, returning the cached value if the same inputs are provided again.“`pythonimport functoolsdef cache_results(func): cache = @functools.wraps(func) def wrapper(*args): if args in cache: return cache[args] result = func(*args) cache[args] = result return result return wrapper@cache_resultsdef expensive_function(n): # Simulate a computationally expensive operation time.sleep(2) return n – nprint(expensive_function(5)) # Takes 2 secondsprint(expensive_function(5)) # Instant – result is cachedprint(expensive_function(10)) # Takes 2 seconds“`This decorator uses a dictionary to store the cached results.
The key is the tuple of input arguments, and the value is the function’s return value.
Performance Comparison: Caching Decorator
The following table compares the execution time of `expensive_function` with and without the caching decorator.
Function Call | Without Caching (seconds) | With Caching (seconds) | Speedup |
---|---|---|---|
expensive_function(5) | 2 | 2 | 1x |
expensive_function(5) | 2 | ~0 | Infinite |
expensive_function(10) | 2 | 2 | 1x |
expensive_function(10) | 2 | ~0 | Infinite |
Note: The “~0” represents near-instantaneous execution due to caching. The actual time will depend on system overhead. The speedup is significantly higher for subsequent calls with the same arguments.
Decorators and Class Methods
Decorators, those elegant little wrappers we use to enhance function behavior, also find a powerful application in the world of class methods. While the underlying principle remains the same – modifying the behavior of a callable – applying decorators to class methods introduces some subtle yet important differences compared to decorating plain functions. Understanding these nuances is key to leveraging the full potential of decorators in object-oriented programming.Decorating class methods allows us to add functionality to methods without modifying their core implementation.
This promotes cleaner code, better separation of concerns, and improved maintainability. We can add logging, input validation, timing measurements, or any other cross-cutting concerns directly to the method’s execution flow, keeping the core logic neatly contained within the method itself.
Decorating Class Methods: A Practical Example
Let’s illustrate with a concrete example. Suppose we have a class representing a bank account, and we want to log every deposit and withdrawal operation. We can achieve this using a decorator:“`pythonimport functoolsdef log_transaction(func): @functools.wraps(func) def wrapper(self,args, –
*kwargs)
result = func(self,
- args,
- *kwargs)
print(f”Transaction: func.__name__
Amount
args[0]”) return result return wrapperclass BankAccount: def __init__(self, balance=0): self.balance = balance @log_transaction def deposit(self, amount): self.balance += amount return self.balance @log_transaction def withdraw(self, amount): if self.balance >= amount: self.balance -= amount return self.balance else: print(“Insufficient funds!”) return self.balanceaccount = BankAccount(100)account.deposit(50)account.withdraw(25)“`This code demonstrates how the `log_transaction` decorator neatly adds logging to both the `deposit` and `withdraw` methods without cluttering their internal logic.
The `functools.wraps` decorator is crucial here; it preserves the original function’s metadata, ensuring that introspection tools (like `help()`) still display the correct information about the decorated method.
Differences Between Decorating Class Methods and Functions
The key difference lies in the `self` parameter. When decorating a class method, the wrapper function must explicitly accept and pass along the `self` parameter, which represents the instance of the class. This is unlike decorating a regular function, where there’s no such implicit first parameter. Failure to handle `self` correctly will lead to errors. Furthermore, the context within a class method decorator is the instance itself, allowing access to instance attributes and methods directly.
Challenges and Solutions in Decorating Class Methods
One potential challenge arises when dealing with methods that use various parameters or have complex signatures. The decorator needs to be flexible enough to handle these variations. Using `*args` and `kwargs` in the wrapper function is the standard solution for handling arbitrary numbers of positional and arguments. Another challenge could be related to error handling. The decorator should ideally handle exceptions gracefully, potentially logging errors or providing alternative responses without disrupting the main functionality of the decorated method.
Proper exception handling within the wrapper function is vital for robust decorator implementation.
A Parameter-Dependent Decorator for Class Methods
Let’s create a decorator that modifies the behavior of a class method based on an input parameter. This example demonstrates conditional logic within the decorator:“`pythonimport functoolsdef conditional_behavior(condition_param): def decorator(func): @functools.wraps(func) def wrapper(self,args, –
*kwargs)
if kwargs.get(condition_param, False): print(f”Conditional logic activated for func.__name__”) # Perform conditional action here kwargs[condition_param] = False # modify the parameter itself return func(self,
- args,
- *kwargs)
else: return func(self,
- args,
- *kwargs)
return wrapper return decoratorclass MyClass: @conditional_behavior(“special_mode”) def my_method(self, value, special_mode=False): return value – 2obj = MyClass()print(obj.my_method(5)) # Output: 10print(obj.my_method(5, special_mode=True)) # Output: Conditional logic activated for my_method, then 10“`This `conditional_behavior` decorator allows us to conditionally change the method’s execution path based on the presence and value of a specific argument.
This offers a flexible way to modify the method’s behavior without changing the core implementation.
Decorators and Metaclasses
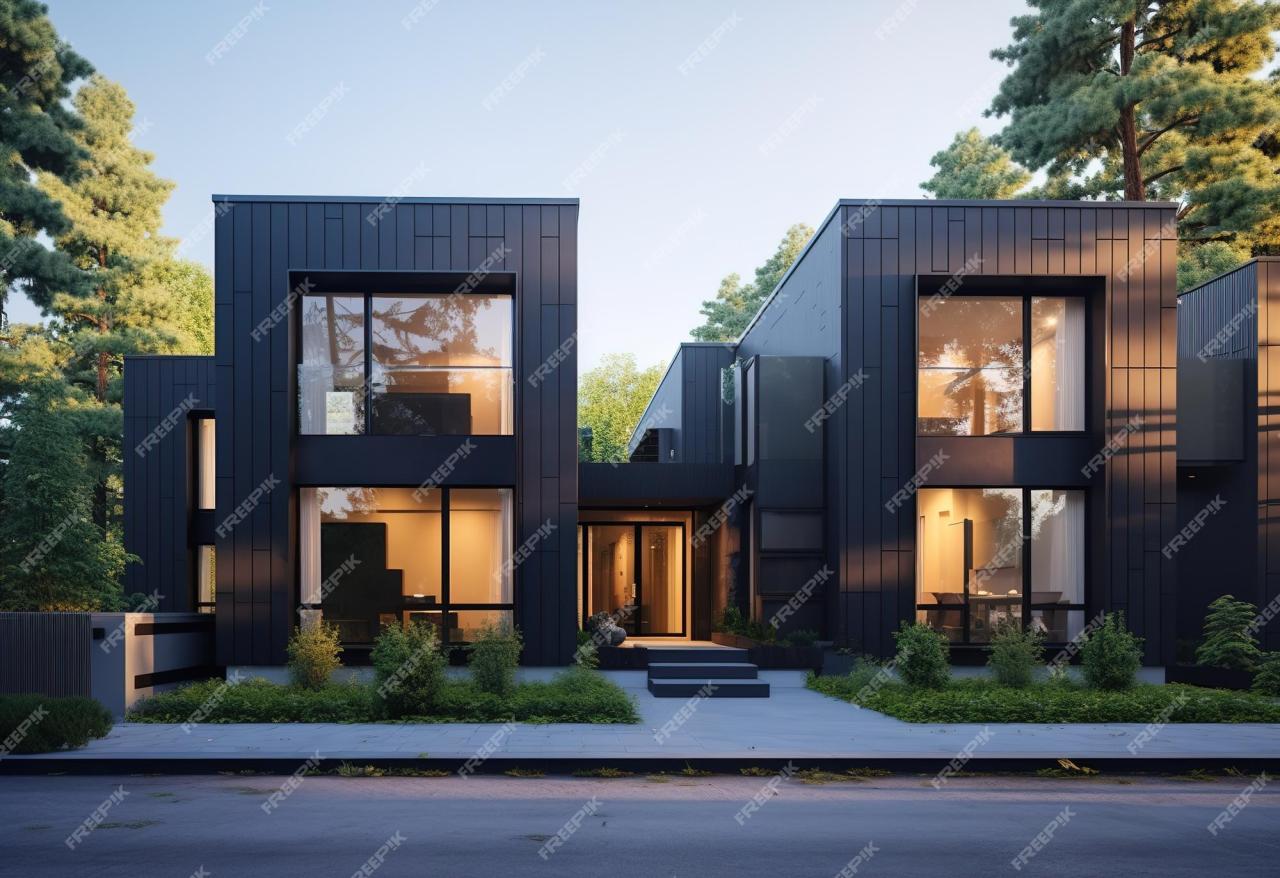
Source: freepik.com
Decorators and metaclasses are powerful tools in Python that allow for dynamic modification of classes and their methods. While seemingly distinct, they share a close relationship, offering complementary ways to achieve similar goals, albeit with different approaches and levels of control. Understanding their interplay unlocks advanced techniques for code organization and flexibility.Metaclasses control the creation of classes, acting as blueprints for classes themselves.
Decorators, on the other hand, modify functions and methods after they’ve been defined. This difference in timing and scope leads to distinct applications and advantages.
Metaclasses Enhance Decorator Functionality
Metaclasses provide a mechanism to apply decorators systematically across an entire class. Instead of individually decorating each method, a metaclass can automatically apply a decorator to all methods during class creation. This reduces boilerplate code and ensures consistent application of the decorator’s logic. For instance, a metaclass could automatically add logging, timing, or access control decorators to all methods within a class, promoting consistency and maintainability.
This centralized approach simplifies the process of adding cross-cutting concerns to multiple methods.
Decorator versus Metaclass Usage Comparison
Choosing between decorators and metaclasses depends on the specific need. Decorators are ideal for modifying individual functions or methods, offering a concise and targeted approach. Metaclasses excel when applying modifications uniformly across all methods of a class, simplifying the implementation and reducing redundancy. Decorators are generally simpler to understand and use for smaller modifications, while metaclasses offer a more powerful, though potentially more complex, solution for large-scale, class-wide transformations.
A practical example might be using a decorator to add logging to a single method for debugging purposes, versus using a metaclass to add logging to all methods for comprehensive system monitoring.
Example Metaclass Adding a Decorator to All Methods, Decorators with a difference
The following example demonstrates a metaclass that adds a simple timing decorator to all methods of a class.“`pythonimport timedef timer(func): def wrapper(*args,
*kwargs)
start = time.time() result = func(*args, – *kwargs) end = time.time() print(f”Function func.__name__ took end – start:.4f seconds”) return result return wrapperclass TimerMeta(type): def __new__(cls, name, bases, attrs): for name, value in attrs.items(): if callable(value): attrs[name] = timer(value) return super().__new__(cls, name, bases, attrs)class MyClass(metaclass=TimerMeta): def method1(self): time.sleep(1) def method2(self, a, b): time.sleep(0.5) return a + bobj = MyClass()obj.method1()result = obj.method2(2, 3)print(f”Result of method2: result”)“`This code defines a `timer` decorator and a `TimerMeta` metaclass.
The `__new__` method iterates through the class attributes. If an attribute is callable (a method), it applies the `timer` decorator. The `MyClass` then uses `TimerMeta` as its metaclass, automatically timing all its methods. The output will show the execution time for each method. This illustrates how a metaclass effectively applies a decorator without explicitly decorating each method individually.
Illustrative Examples
Decorators, while powerful, can sometimes seem abstract. Seeing them in action, however, illuminates their true value. The following examples showcase how decorators enhance code readability, maintainability, and even enable advanced programming paradigms. We’ll move beyond simple logging examples and delve into scenarios where the benefits become truly apparent.
Improved Code Readability with Decorators
Imagine a function that needs to perform authentication, logging, and error handling. Without decorators, this function would quickly become cluttered and difficult to read. Let’s consider a hypothetical scenario of processing user requests for a sensitive resource. The core functionality is to process the request, but we need to ensure the user is authenticated, log the request details, and handle potential exceptions.
A decorator elegantly separates these concerns. The core logic remains clean and focused, while the decorator handles the cross-cutting concerns.
Impact of Decorators on Code Maintainability
Let’s say we have a system where multiple functions require authentication. Without decorators, we’d need to repeat the authentication logic in each function. This leads to redundancy and makes maintenance a nightmare. If the authentication process changes, we have to modify every single function. A decorator, however, encapsulates the authentication logic in one place.
Changes to the authentication process only require modification in the decorator, significantly improving maintainability. Imagine needing to add multi-factor authentication – a decorator makes this change localized and straightforward.
Visual Representation of Decorator Execution Flow
Consider a simple function `process_data()` that takes some input and returns a result. Without Decorator:“`process_data(input) –> [processing logic] –> result“` With a Logging Decorator:“`process_data(input) –> [decorator: log start] –> [processing logic] –> [decorator: log end] –> result“`The decorator intercepts the call to `process_data()`, executes its own logic (logging in this case) before and after the original function, and then passes control back to the original function.
This visual representation clearly demonstrates how the decorator extends the functionality without modifying the core logic of the original function.
Implementing Aspect-Oriented Programming with Decorators
Aspect-oriented programming (AOP) focuses on separating cross-cutting concerns from the core business logic. Decorators are a powerful tool for implementing AOP concepts. Let’s consider a scenario where we want to time the execution of multiple functions. We can create a decorator that measures the execution time of any function it decorates. This timing aspect is separated from the core functionality of the individual functions.
The decorator cleanly adds the timing aspect without cluttering the main functions’ code. This is a classic example of AOP, where concerns like logging, security, and timing are handled separately from the main application logic, resulting in cleaner, more maintainable code.
Advanced Decorator Patterns and Best Practices
Decorators are a powerful tool in Python, but their true potential unfolds when we delve into advanced techniques and best practices. Mastering these allows us to create more robust, flexible, and maintainable code. This section explores parameterized decorators, exception handling within decorators, and crucial best practices for writing effective decorator functions. We’ll also highlight common pitfalls to avoid, ensuring your decorators enhance, not hinder, your projects.
Parameterized Decorators
Parameterized decorators extend the functionality of basic decorators by allowing you to pass arguments to modify their behavior. This dynamic nature makes them incredibly versatile. Instead of a fixed transformation, a parameterized decorator can tailor its actions based on the input parameters. A simple example would be a decorator that caches the results of a function based on a specific input; the caching strategy could be altered via parameters.
This allows for different caching mechanisms (like LRU cache with a configurable size) without needing separate decorator functions for each. To create a parameterized decorator, you essentially wrap the inner decorator function within another function that takes the parameters. This outer function then returns the inner decorator, which ultimately wraps the original function.
Exception Handling within Decorators
Robust decorators anticipate potential errors and handle them gracefully. Failing to do so can lead to unexpected crashes or masked exceptions, making debugging difficult. A well-designed decorator should include `try…except` blocks to catch specific exceptions, log errors appropriately, and potentially return a default value or raise a custom exception to indicate the failure. Ignoring exceptions can lead to silent failures, making it harder to track down problems in the decorated function.
So, you’re thinking about decorators with a difference? Maybe something bold, something unexpected? To really get your ideas out there, you need the right platform, and that’s where understanding video marketing comes in. Check out this great guide on getting it on with youtube to see how you can boost your reach. Then, armed with that knowledge, you can really make those unique decorator ideas shine online!
Proper exception handling provides a layer of protection, ensuring the application remains stable even if the decorated function encounters unforeseen issues.
Best Practices for Writing Clear, Maintainable, and Reusable Decorators
Writing effective decorators requires attention to several key aspects. First, use descriptive names that clearly communicate the decorator’s purpose. Second, strive for single responsibility: each decorator should have one clear, well-defined task. Third, use docstrings to clearly explain the decorator’s function, parameters, and expected behavior. Fourth, favor simplicity and readability; avoid overly complex logic within the decorator.
Fifth, ensure the decorator doesn’t introduce unintended side effects. By following these guidelines, you create decorators that are easy to understand, reuse, and maintain, promoting better code organization and collaboration.
Common Pitfalls to Avoid When Working with Decorators
Several common mistakes can lead to unexpected behavior or difficult-to-debug code. One is forgetting to return the wrapped function from the decorator, which will render the decorator ineffective. Another is improper handling of arguments, particularly arguments, when dealing with parameterized decorators or decorators that need access to the function’s arguments. A third pitfall is neglecting exception handling, as discussed previously.
Finally, overusing decorators can lead to overly complex and hard-to-understand code. A well-designed decorator enhances readability; poorly designed decorators can obscure it. Careful consideration of these points is essential for effective decorator implementation.
End of Discussion
From basic functionality to advanced techniques, we’ve explored the versatile world of Python decorators. By understanding decorator factories, chaining, and their application in diverse scenarios – from logging and validation to caching and even metaclasses – you’re now equipped to write cleaner, more efficient, and maintainable code. Remember, mastering decorators isn’t just about writing efficient code; it’s about crafting elegant and readable solutions that stand the test of time.
So go forth and decorate!
FAQ Corner
Can I use decorators with asynchronous functions?
Yes! You can use decorators with asynchronous functions (using `async` and `await`). Just ensure your decorator is also an asynchronous function using the `async` .
What happens if a decorator raises an exception?
Exceptions raised within a decorator will propagate up to the caller of the decorated function, unless explicitly handled within the decorator itself using a `try…except` block.
Are decorators only useful for small functions?
No, decorators can be applied to functions of any size. Their benefits become increasingly apparent as the complexity of your functions grows, helping to manage and organize logic.
How do decorators affect performance?
Well-written decorators have minimal performance impact. However, poorly implemented decorators, particularly those with significant internal logic, can introduce overhead. Profiling your code is recommended if performance is a major concern.